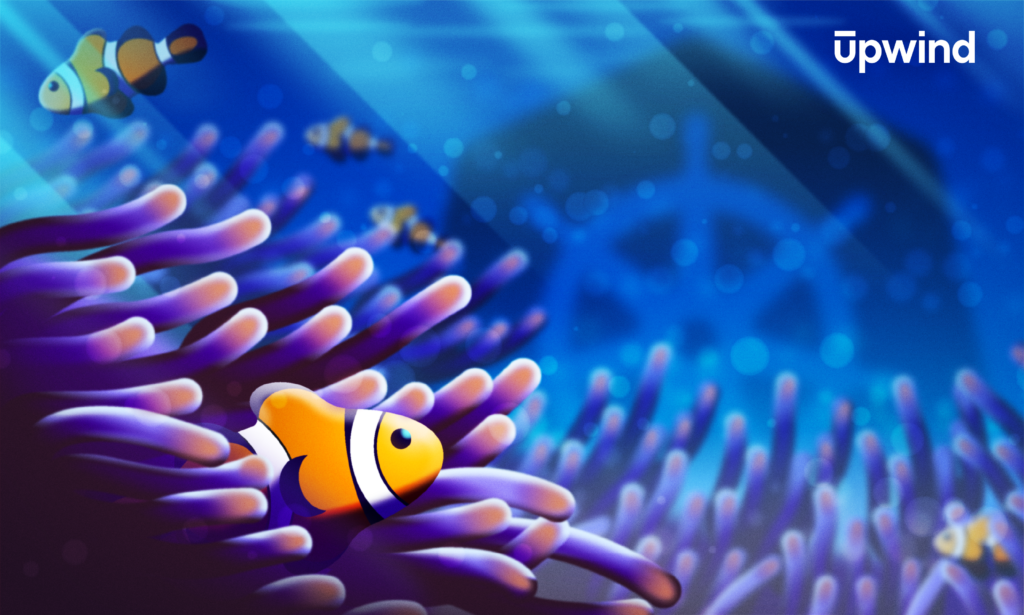
When it comes to Kubernetes, managing identities is pivotal for ensuring secure and efficient cluster operations. These identities can be human users or machines, each requiring specific permissions to perform their tasks. In our latest research, we have explored what Kubernetes identities are, the default identities, the permissions they can have, how to configure these permissions, common misconfigurations, and the authentication and authorization mechanism.
What are Kubernetes Identities?
In Kubernetes, identities are used to authenticate and authorize interactions within the cluster. These identities can represent:
- User Identities: Individuals who interact with the cluster through the Kubernetes API,
kubectl
, or the Kubernetes dashboard, mostly integrated through Kubernetes providers like the big cloud providers. - Machine Identities: Components such as nodes, pods, and controllers that need permissions to operate within the cluster.
User Identities
Managing user identities involves controlling access and permissions for users who interact with the cluster. Kubernetes doesn’t manage users directly. Instead, it integrates with external identity providers and leverages Role-Based Access Control (RBAC) to define and enforce permissions.
Machine Identities
In Kubernetes, machine identities are represented as Service Accounts. Kubernetes has pre-configured service accounts:
- Default: Each namespace in Kubernetes has a default service account automatically created. Any pod that does not explicitly specify a service account will use this default service account.
- Controller Identities: Controllers monitor the state of Kubernetes objects and make changes to bring the actual state closer to the desired state.
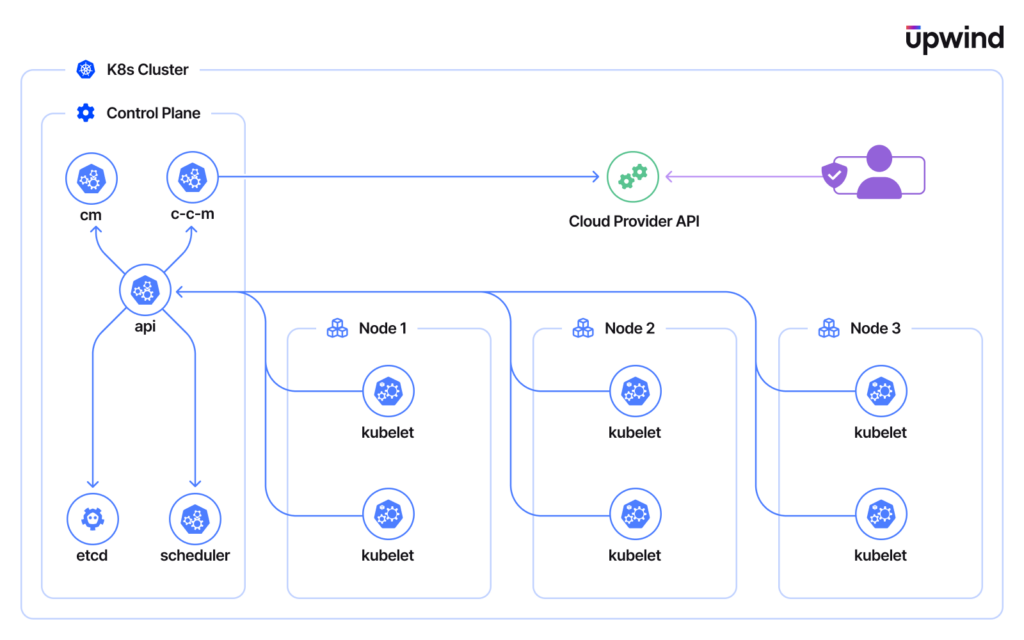
Authentication and Authorization
Authentication verifies the identity of users accessing the Kubernetes API. Kubernetes supports several authentication methods:
- X.509 Client Certificates: Users can authenticate using client certificates. These certificates are typically issued by a certificate authority (CA) and configured in the
kubeconfig
file used bykubectl
. - Static Token File: Users can authenticate using static tokens listed in a file. This is suitable for simple or temporary setups.
- Webhook Token Authentication: This method allows integration with external authentication systems via a webhook.
- OpenID Connect (OIDC): OIDC is a common method for integrating with identity providers like Google, AWS Cognito, or any OIDC-compliant provider. This setup allows for single sign-on (SSO) and centralized user management.
Authorization ensures that the user has the necessary permissions to perform the requested action. Kubernetes provides several built-in authorization modes, each catering to different needs and environments:
- Attribute-Based Access Control (ABAC): Uses policies defined in a file to control access based on user attributes.
- Role-Based Access Control (RBAC): The most commonly used authorization mode, based on roles and role bindings.
- Webhook: Allows integrating with external authorization services.
Kubernetes Service Accounts in the Cloud
In addition to authentication and authorization, you can also give your Kubernetes service accounts access to use cloud resources securely. This is achieved by binding a cloud identity (such as an IAM Role in AWS, Workload Identity in GCP, or Managed Identity in Azure) to the Kubernetes service account. When a pod uses this service account, it can request temporary credentials from the cloud provider. These temporary credentials allow the pod to access cloud resources, without needing to use long-lived credentials.
How It Works:
- Bind Cloud Identity: Associate a cloud identity (e.g., IAM Role in AWS) with a Kubernetes service account.
- Request Temporary Credentials: The pod uses the Kubernetes service account token to request temporary credentials from the cloud provider.
- Access Cloud Resources: With the obtained temporary credentials, the pod can access cloud resources.
Given RBAC’s important role in enforcing authorization within Kubernetes, it’s essential to understand its workings. This core mechanism ensures robust security and governance. Let’s delve into how RBAC operates.
Role-Based Access Control
Roles
and ClusterRoles
define sets of permissions for accessing Kubernetes resources. These permissions are then assigned to users or service accounts through RoleBindings
and ClusterRoleBindings
. While both Roles
and ClusterRoles
serve similar functions, they differ in scope.
- Roles: Define permissions within a specific namespace. A
Role
contains rules that specify permitted actions on resources within that namespace.- Scope: Namespace-specific.
- Use Case: Restricting access to resources within a particular namespace, such as limiting what actions developers can perform within the development namespace.
- ClusterRoles: Define permissions that apply cluster-wide. Unlike
Roles
,ClusterRoles
can be used to grant access to:- Resources in all namespaces.
- Non-namespaced resources (e.g., nodes, persistent volumes).
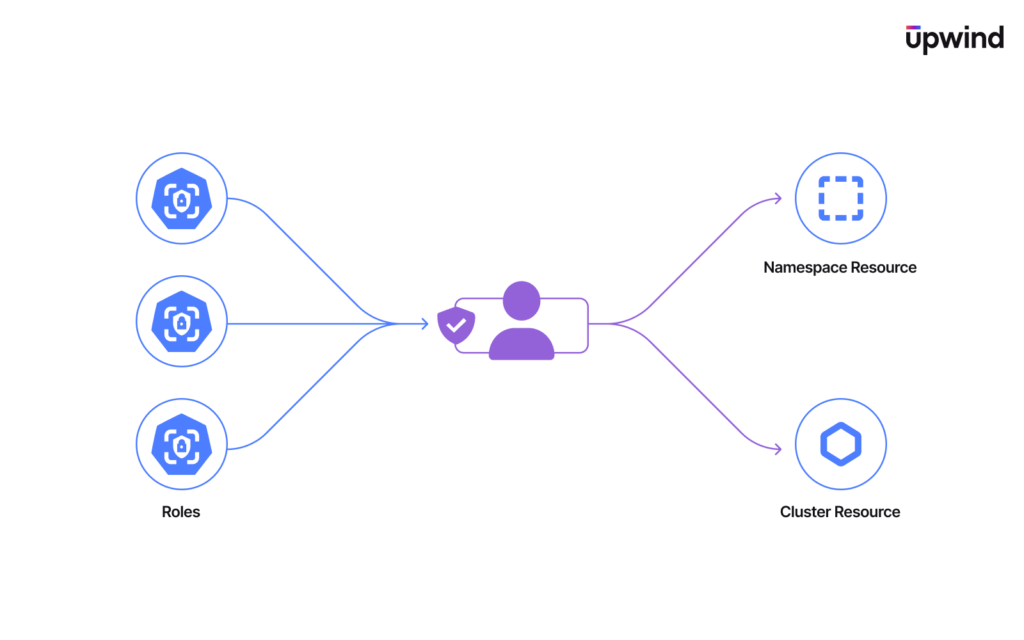
RBAC permissions in Kubernetes are defined by a combination of a resource and a verb. The resource refers to any Kubernetes object, while the verb specifies the action that can be performed on that resource. Common verbs include:
get
: Grants permission to retrieve the specified resourcelist
: Grants permission to list all instances of the specified resourcecreate
: Grants permission to create a new instance of the specified resourceupdate
: Grants permission to update an existing instance of the specified resourcepatch
: Grants permission to partially update an existing instance of the specified resourcedelete
: Grants permission to delete the specified resourcewatch
: Grants permission to watch for changes to the specified resource. This is often used by controllers to stay updated on resource changes.
there are additional verbs:
proxy
: Grants permission to access the proxy subresource of the specified resource. This is used for accessing services through the Kubernetes proxydeletecollection
: Grants permission to delete a collection of resourcesbind
: Grants permission to assign roles to other users, groups, or service accounts by creating or modifyingRoleBindings
andClusterRoleBindings
.escalate
: Grants permission to create a binding to a role that is more privileged than any currently owned (e.g., a user with this verb can escalate their permissions by binding themselves to a more privileged role).impersonate
: Grants permission to impersonate other service accounts
Resources in Kubernetes are the various types of objects that can be managed within the cluster. Each resource belongs to a specific API group and version. Resources represent different aspects of the cluster’s state and configuration. some of the core resources are:
- Pods: The smallest and simplest Kubernetes object. A Pod represents a set of running containers on your cluster.
- Nodes: Represents a single worker machine in the cluster.
- Secrets: Stores sensitive information, such as passwords,
OAuth
tokens, and SSH keys. - Namespaces: Provides a mechanism for isolating groups of resources within a single cluster.
- Custom Resource Definitions (CRD): These are resources defined by users or third-party applications to extend the Kubernetes API.
To get a full list of resources supported in your cluster you can use kubectl
:
kubectl api-resources
Copied
API groups in Kubernetes are a way to group together related APIs. This grouping helps to organize the large number of APIs and makes it easier to extend the Kubernetes API. Each API group is represented by a specific path in the Kubernetes API URL.
- Core API Group: This is the default API group and includes core resources like Pods, Services, and Namespaces. These resources are accessible via the
/api/v1
path. - Named API Groups: These are additional API groups that contain other resources and are accessible via
/apis/<group>/<version>
. API groups include: - apps: Contains resources related to applications, such as Deployments,
StatefulSets
, andDaemonSets
. Accessible via/apis/apps/v1
. - batch: Contains resources for batch processing, like
Jobs
andCronJobs
. Accessible via/apis/batch/v1
. - rbac.authorization.k8s.io: Contains resources for role-based access control, such as
Roles
,ClusterRoles
,RoleBindings
, andClusterRoleBindings
. Accessible via/apis/rbac.authorization.k8s.io/v1
.
- apps: Contains resources related to applications, such as Deployments,
Configuring Permissions
Permissions are configured using Roles
and RoleBindings
. Here’s an example:
Step 1: Define a Role
A Role
defines permissions within a namespace.
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: pod-reader
rules:
— apiGroups: [""]
resources: ["pods"]
verbs: ["get", "watch", "list"]
Copied
Step 2: Bind the Role
to a Service Account
A RoleBinding
assigns the defined Role
to a specific service account.
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods
namespace: default
subjects:
- kind: ServiceAccount
name: default
namespace: default
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
Copied
Authentication and Authorization Flow
The authentication and authorization flow in Kubernetes involves verifying the identity of users or entities attempting to interact with the cluster and determining what actions they are allowed to perform. Here’s a detailed explanation of each step in this process:
- API Request: A user, service, or application makes a request to the Kubernetes API server (e.g.,
kubectl
apply
,kubectl get
, etc.). - Authentication:
- The API server receives the request and checks the authentication information (e.g., client certificates, bearer token).
- The API server verifies the identity of the requester based on the provided authentication method.
- Authorization:
- Once authenticated, the API server checks if the requester is authorized to perform the requested action.
- The authorization mechanism checks if the requester has the necessary permissions.
- For RBAC, the API server checks role bindings and cluster role bindings to determine if the requester’s roles grant the required permissions for the action on the specified resources.
- Admission Control:
- Admission Control intercepts requests to the Kubernetes API before they are persisted as objects in the cluster.
- Admission controllers validate, mutate, or reject requests based on configured policies, ensuring that only allowed requests proceed.
- Examples of admission controllers include
NamespaceLifecycle
,ResourceQuota
,PodSecurityPolicy
, and custom controllers.
- Request Execution:
- If the request passes authentication, authorization, and admission control, it is executed.
- The API server processes the request and interacts with the relevant Kubernetes resources (e.g., creating a pod, updating a deployment).
- Response:
- The API server sends a response back to the requester with the outcome of the request (success or error).
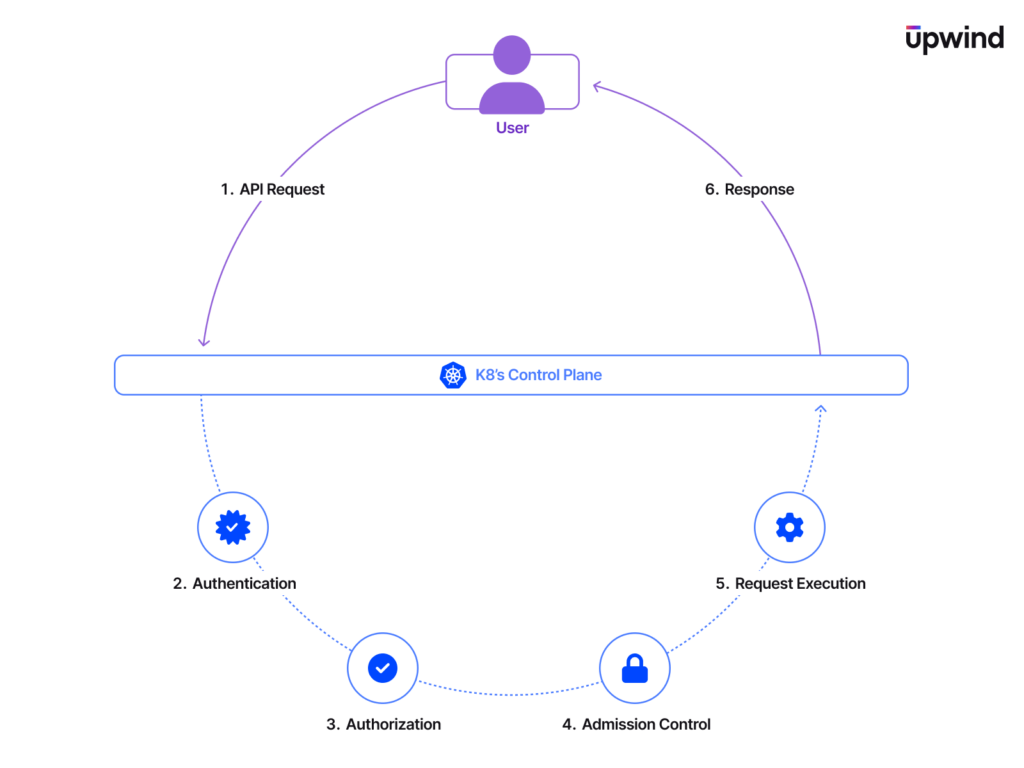
Possible Misconfigurations in Kubernetes Identities
While Kubernetes provides robust mechanisms for managing identities and access control through RBAC (Role-Based Access Control) and service accounts, misconfigurations can inadvertently expose clusters to security risks. Here are some common misconfigurations to be aware of:
- Over-Privileged Roles and Role Bindings:
- Issue: Assigning roles with more permissions than necessary (
ClusterRole
orRole
) to users or service accounts. - Risk: Users or applications may have excessive privileges, potentially leading to unauthorized access or unintended actions within the cluster.
- Mitigation: Follow the principle of least privilege, regularly audit roles and role bindings, and use namespace-scoped roles where possible to limit exposure.
- Issue: Assigning roles with more permissions than necessary (
- Excessive Use of Cluster-Admin Role:
- Issue: Assigning the
cluster-admin
role to users or service accounts unnecessarily. - Risk: Users granted
cluster-admin
have unrestricted access across the entire Kubernetes cluster, posing a significant security risk if credentials are compromised. - Mitigation: Reserve
cluster-admin
privileges for system administrators only. Use RBAC to define granular roles tailored to specific operational needs.
- Issue: Assigning the
- Multiple Service Accounts in the Same Namespace:
- Issue: Configuring multiple service accounts for different pods within the same namespace without proper isolation.
- Risk: Pods may communicate with each other, potentially leading to unauthorized access and secret leakage if one pod is compromised.
- Mitigation: Use network policies to control inter-pod communication. Regularly audit service account usage and segregate sensitive workloads into different namespaces.
- Excessive Permissions Granted to Service Accounts by Third-Party Tools:
- Issue: Implementing tools within the cluster that create service accounts with excessive permissions for their operations.
- Risk: These service accounts can introduce security vulnerabilities if they have more privileges than necessary, leading to potential exploitation.
- Mitigation: Carefully review and configure the permissions of service accounts created by third-party tools. Limit their privileges to the minimum required for operation and regularly audit these accounts.
- Over-Permissive Kubernetes Service Accounts with Cloud Resource Access
- Issue: Kubernetes service accounts configured with permissions extending beyond the cluster to cloud resources, including permissions not aligned with the service account’s intended purpose.
- Risk: Over-permissive service accounts can be exploited to gain unauthorized access to cloud resources, potentially leading to data breaches, resource manipulation, and infrastructure compromise.
- Mitigation: Enforce the principle of least privilege by granting service accounts only the permissions necessary for their specific tasks. Regularly audit service account permissions and ensure that access to cloud resources is tightly controlled and monitored. Use Kubernetes RBAC (Role-Based Access Control) and cloud IAM (Identity and Access Management) policies to minimize exposure and reduce the attack surface.
Kubernetes resources define the desired state of the cluster. User and machine identities have specific permissions to change or monitor these resources using the appropriate API groups. Each identity is granted permissions tailored to its needs. For example, controllers use their identity and assigned permissions to watch for changes in resources. When a change is detected, the controller will apply the necessary adjustments to the environment.
Enhancing Kubernetes Security with Upwind: Mitigating Risks and Elevating Visibility
In a Kubernetes environment, understanding and managing identities is crucial for maintaining security and ensuring smooth operations. Upwind features advanced Identity Security designed to provide comprehensive visibility and control over Kubernetes identities.
Upwind Identity Security offers the following capabilities:
- Complete Identity Inventory: Gain a holistic view of all Kubernetes identities within your environment. This includes both user and service accounts, allowing you to see who and what has access to your cluster.
- Detailed Permission Mapping: Understand the exact permissions each identity holds. Upwind maps out permissions for every identity, helping you quickly identify over-permissive roles and potential security risks.
- Deployment Visibility: Track where each identity is deployed across your Kubernetes cluster. This ensures that you are aware of identity usage patterns and can detect any unauthorized access or unusual activity.
By leveraging Upwind’s identities module, you can mitigate risks associated with over-privileged identities, prevent potential security breaches, and maintain a high level of visibility and control over your Kubernetes environment. This proactive approach to identity management helps secure your cluster and ensures that only the necessary permissions are granted to each identity.
Learn More
To learn more about Upwind Identity Security for Kubernetes, visit the Upwind Documentation Center (login required) or schedule a demo.